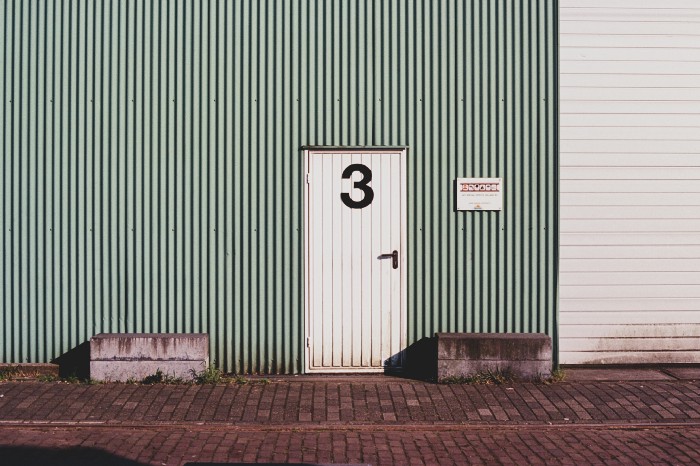
Daaps Without Metamask
Mehrad Sadeghi • November 12, 2018
So I developed my dapps based on Ethereum and Solidity for back-end and React.js for front-end, And I used Metamask as the wallet to manage accounts.
Finally, I got to the point that I couldn’t make users to install a separate wallet or an extension in their browser, in order to interact with my contracts.
Now I want to show you how to sign transactions with your private key and send them into the network via web3.js.
Contract Deployment:
web3.js
file:
const Web3 = require('web3');
// your network address, for example for rinkeby network :
const networkAddress = 'https://rinkeby.infura.io/v3/your-token';
const provider = new Web3.providers.HttpProvider(networkAddress);
const web3 = new Web3(provider);
module.exports = web3;
deploy.js
file :
const web3 = require('./web3');
const privateKey = 'your private key';
.
.
.
contract = await new web3.eth.Contract(
JSON.parse(compiledContract.abi)
).deploy({ data: `0x${compiledContract.bytecode}` });
const options = {
data: contract.encodeABI(),
gas: '1000000'
};
let signedTransaction = await web3.eth.accounts.signTransaction(options, privateKey);
const deploy = await web3.eth.sendSignedTransaction(signedTransaction.rawTransaction);
console.log(
"Address of deployed contract : ", deploy.contractAddress
);
Send Transaction:
Same as deploying a contract, except that you have to specify the address of the contract you’re sending the transaction to. So the options
object will be :
const options = {
to: 'target contract address',
data: contract.encodeABI(),
gas: '1000000'
};
Hope this article helpes you with your blockchain developments.
You can find one of my open source projects on Github and its interactive dapp in here.